Nana is a "cross-platform library for GUI programming in modern C++ style". More information is available on this website
http://nanapro.org/en-us/.
I wanted to use this library with a simple C++ application on Ubuntu. So I created a
CMake project with the following folders (see the screenshot below).
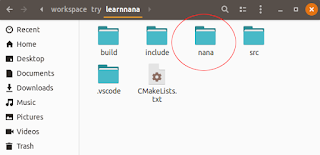
Following the
getting started and
installation instructions here
http://nanapro.org/en-us/blog/2016/05/an-introduction-to-nana-c-library/, I downloaded the
nana source files, placed them under the
nana folder and compiled them into the static library
libnana.a.
Next, I created a simple
Hello World C++ source file and placed under the
src folder.
I then created a
CMakeLists.txt file that describes the project and dependencies.
cmake_minimum_required(VERSION 2.8.6)
project(runnana)
find_package (Threads REQUIRED)
find_package (X11 REQUIRED)
find_package(Freetype REQUIRED)
set (CMAKE_BUILD_TYPE Debug)
set (LIBRARY_OUTPUT_PATH bin)
set (CMAKE_CXX_STANDARD 14)
set (CMAKE_CXX_STANDARD_REQUIRED ON)
set (CMAKE_ARCHIVE_OUTPUT_DIRECTORY ./bin)
set (CMAKE_LIBRARY_OUTPUT_DIRECTORY ./bin)
set (CMAKE_RUNTIME_OUTPUT_DIRECTORY ./bin)
set (NANA_INCLUDE nana/include)
set (NANA_LINK nana/build/bin)
include_directories (
${FREETYPE_INCLUDE_DIRS}
${X11_INCLUDE_DIR}
${NANA_INCLUDE}
)
link_directories (
${NANA_LINK}
)
set (RUNNANA_LINK_LIBS
stdc++fs
nana
${X11_LIBRARIES}
${CMAKE_THREAD_LIBS_INIT}
rt
Xft
png
asound
fontconfig
${FREETYPE_LIBRARIES}
)
set (RUNNANA_INCLUDE
include
)
file (GLOB RUNNANA_SOURCES "src/*.c*")
add_executable (runnana ${RUNNANA_SOURCES})
target_include_directories (runnana PUBLIC ${RUNNANA_INCLUDE})
target_link_libraries (runnana ${RUNNANA_LINK_LIBS} stdc++fs)
Using this
CMake file, I can easily build the project and compile the simple hello world executable, as shown below.
The example hello world application.