- To run this web app, go to http://dominoc925pages-appspot.com/webapp/watermark_image.html.
- Optional. Click Settings and change the Watermark text, font style, size, color, transparency, location, alignment, scale and other properties.
- Simply drag and drop the image files (JPEG, PNG, GIF) into the dashed box. Or click the Choose Files button and select one or more image files.
The image(s) are watermarked.
- Just right click on the image and choose Save image as to save out the watermarked image.
Monday, November 26, 2012
Batch watermark images with this HTML5 web app
There are a few online web sites that can place watermarks on one or many images. However, in order to use those services, the images need to be uploaded to the web server before the water marking process can be done. The canvas element of HTML5 is a rich object for image processing and I used this element to write a web app to perform a similar water marking task locally using a modern browser like Chrome and Firefox or Internet Exporer 10+ without having to upload to a web server.
Monday, November 19, 2012
GeoMedia C# code snippet to get the warehouse connection's table list
It is useful to programmatically retrieve the list of tables in a GeoMedia warehouse via the connection object. The GeoMedia MetadataService can be called upon to generate the list in a String array. The following C# code snippet shows how this can be done.
The connection object is passed in to the GetTableList method and returns an ArrayList of table names.
The connection object is passed in to the GetTableList method and returns an ArrayList of table names.
using GeoMedia = Intergraph.GeoMedia.GeoMedia;
using PCSS = Intergraph.GeoMedia.PCSS;
using PBasic = Intergraph.GeoMedia.PBasic;
using GDO = Intergraph.GeoMedia.GDO;
using PClient = Intergraph.GeoMedia.PClient;
using PService = Intergraph.GeoMedia.PService;
using GMService = Intergraph.GeoMedia.GMService;
//...etc...
private ArrayList GetTableList(PClient.Connection conn)
{
String[] list = null;
ArrayList tables = new ArrayList();
object oConn = (object)conn;
int mask = 0;
object vTables = null;
GMService.MetadataService metadataSvc = null;
//Create an instance of the GeoMedia MetadataService
metadataSvc = new GMService.MetadataService();
//Pass in the connection object to the MetadataService
metadataSvc.set_Connection(ref oConn);
//Get the connection's list of tables and put them into the vTables array
metadataSvc.GetTables(ref mask, ref vTables);
//copy the table list into an ArrayList and return to the calling function
list = (String [])vTables;
for (int i = 0; i < list.Length; i++)
tables.Add(list[i].ToUpper());
return tables;
}
Monday, November 12, 2012
Copy point database attributes to polygons in Global Mapper
A common task in GIS is to copy the attributes of points to the enclosing area polygons. It is simple to perform the task in Global Mapper as illustrated below.
- Start Global Mapper. Load a polygon layer and a point layer.
- Press ALT+D.
The Digitizer tool is activated. - Click and drag a rectangle around the polygons and points.
The polygons and points are selected. - Press the mouse right button anywhere in the map view.
A pop up menu appears. - Select Attribute Functions | Add attributes to Selected Areas from Points.
The following message appear. - Click Yes. Then click OK.
The point attributes are copied to the polygons.
Monday, November 5, 2012
2-D equations to transform between world and local coordinate systems
The geographic (world) coordinate systems e.g. UTM typically have the positive Y-axis pointing upwards and the X-axis pointing eastward. Local coordinate systems such as the HTML5 or SVG canvas have the positive Y-axis pointing downwards. The figure below shows the differences between the world and local coordinate systems.
I found it useful to work out the 2-D equations to transform coordinates between the world and the local coordinate systems for encoding into software programming functions.
A simple non-matrix example is show below.
In the figure below, an image file has the origin O with the world coordinates (x0,y0) and dimensions of Δx and Δy.
The corresponding image file in the local coordinate system has the origin O'(x0',y0') and dimensions of Δx' and Δy'. Typically (x0',y0') = (0,0).
A point P(x,y) in the world coordinate system can be transformed to a point P'(x',y') in the local coordinate system with the following equations:
x' = (x - x0) * Sx
y' = -(y - y0) * Sy
where
Sx = Δx'/Δx
Sy = Δy'/Δy
A point P'(x',y') in the local coordinate system can be transformed to a point P(x,y) in the world coordinate system with the following equations:
x = (x'/Sx) + x0
y = -(y'/Sy) + y0
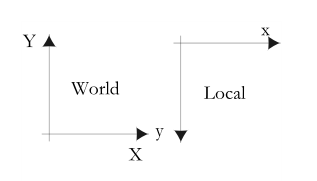
I found it useful to work out the 2-D equations to transform coordinates between the world and the local coordinate systems for encoding into software programming functions.
A simple non-matrix example is show below.
In the figure below, an image file has the origin O with the world coordinates (x0,y0) and dimensions of Δx and Δy.
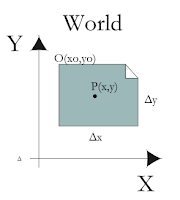
The corresponding image file in the local coordinate system has the origin O'(x0',y0') and dimensions of Δx' and Δy'. Typically (x0',y0') = (0,0).
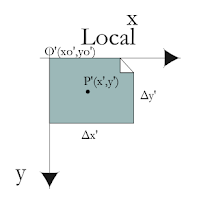
A point P(x,y) in the world coordinate system can be transformed to a point P'(x',y') in the local coordinate system with the following equations:
x' = (x - x0) * Sx
y' = -(y - y0) * Sy
where
Sx = Δx'/Δx
Sy = Δy'/Δy
A point P'(x',y') in the local coordinate system can be transformed to a point P(x,y) in the world coordinate system with the following equations:
x = (x'/Sx) + x0
y = -(y'/Sy) + y0
Subscribe to:
Posts (Atom)