Down the Raspberry Pi build tools
The first thing is to get the Raspberry cross compilation tools from the git repository.
In Ubuntu, open a Terminal. Type in the following to download the tools to a folder e.g. /path/to/programs/pi/.
$ cd ~/programs/pi/
$ git clone https://github.com/raspberrypi/tools.git
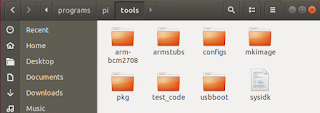
Create a CMake toolchain file
Next, create a cmake file e.g. /path/to/workspace/toolchain-rpi.cmake specifying the correct Raspberry cross compilation tools for compiling C/C++ source files with the CMAKE_C_COMPILER and CMAKE_CXX_COMPILER macros. Also the root folder of the Raspberry Pi libraries and include files need to be specified with the CMAKE_FIND_ROOT_PATH macro.
Choose the right ones for your Raspberry Pi chipset.
Listing of toolchain-rpi.cmake
SET(CMAKE_SYSTEM_NAME Linux) SET(CMAKE_SYSTEM_VERSION 1) # define the C cross compiler for the Raspberry Pi SET(CMAKE_C_COMPILER $ENV{HOME}/programs/pi/tools/arm-bcm2708/arm-rpi-4.9.3-linux-gnueabihf/bin/arm-linux-gnueabihf-gcc) # define the C++ cross compiler for the Raspberry Pi SET(CMAKE_CXX_COMPILER $ENV{HOME}/programs/pi/tools/arm-bcm2708/arm-rpi-4.9.3-linux-gnueabihf/bin/arm-linux-gnueabihf-g++) # define the root location of the Raspberry Pi libraries and includes SET(CMAKE_FIND_ROOT_PATH $ENV{HOME}/programs/pi/tools/arm-bcm2708/arm-linux-gnueabihf/arm-linux-gnueabihf/sysroot) SET(CMAKE_FIND_ROOT_PATH_MODE_PROGRAM NEVER) SET(CMAKE_FIND_ROOT_PATH_MODE_LIBRARY ONLY) SET(CMAKE_FIND_ROOT_PATH_MODE_INCLUDE ONLY) add_definitions(-Wall -std=c11)
Create the workspace
In the same example project workspace folder /path/to/workspace/, create two folders animallib and uselib, the first for the library files, and the later for the executable files.
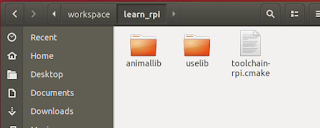
Create the static library
The next step is to create the header file and define the classes that will be used by the example executable. Place the Animal.h and Animal.cpp files respectively in the include and src folders under the /path/to/workspace/animallb/ folder.
Listing of Animal.h
#include <string> using namespace std; class Animal { private: string name; public: Animal(string); virtual void print_name(); };
Listing of Animal.cpp
#include <iostream> #include "Animal.h" using namespace std; Animal::Animal(string name): name (name) {} void Animal::print_name(){ cout << "Name is " << this->name << endl; }
Create the static library's CMakeLists.txt file in the animallib folder e.g. /path/to/workspace/animallib/. As defined, this will help to generate a static library libanimallib.a and a header file animallib_Export.h.
cmake_minimum_required(VERSION 2.8.6) project (animallib) set (BUILD_SHARED_LIBS OFF) set (CMAKE_BUILD_TYPE Debug) #include *.h files under the include folder and #the project's output folder e.g. build include_directories (include ${PROJECT_BINARY_DIR}) #compile all *.cpp source files under the src folder file (GLOB SOURCES "src/*.cpp") #output static library export file *.a and #output macro definitions include file include (GenerateExportHeader) add_library(animallib STATIC ${SOURCES}) GENERATE_EXPORT_HEADER (animallib BASE_NAME animallib EXPORT_MACRO_NAME animallib_EXPORT EXPORT_FILE_NAME animallib_Export.h STATIC_DEFINE animallib_BUILT_AS_STATIC )
Open up a Terminal and change directory to the static library's folder e.g. /path/to/workspace/animallib/.
If the build folder does not exist, create it.
$ mkdir build
$ cd build
Type in the cmake command with the CMAKE_TOOLCHAIN_FILE variable pointing to the previously created Raspberry Pi toolchain CMake file.
$ cmake -D CMAKE_TOOLCHAIN_FILE=/path/to/workspace/toolchain-rpi.cmake ..
![]() | ||
Build files are generated |
In the Terminal, type in the make command to generate the static library file.
$ make
![]() |
Static library compilation messages |
Now that the library has been generated, the next thing is to use the library's classes and functions in an executable e.g. uselib project under /path/to/workspace/uselib/.
Listing of uselib.cpp
#include "Animal.h" #include "animallib_Export.h" int main(int argc, char *argv[]){ Animal animal("Dog"); animal.print_name(); return (0); }
Place the source code file uselib.cpp under the src directory e.g. /path/to/workspace/uselib/src/.
Then create a CMakeLists.txt file under the /path/to/workspace/uselib/ folder.
cmake_minimum_required(VERSION 2.8.6) project (UseLib) # link as a static library set(CMAKE_EXE_LINKER_FLAGS "-static") set (EXAMPLE_DIR $ENV{HOME}/Documents/workspace/learn_rpi/) # name and location of the library to link with the executable set (PROJECT_LINK_LIBS animallib) link_directories (${EXAMPLE_DIR}/animallib/build/) include_directories ( ${EXAMPLE_DIR}/animallib/include ${EXAMPLE_DIR}/animallib/build ) #compile all *.cpp source files under src folder file (GLOB SOURCES "src/*.cpp") #output executable name as uselib add_executable (uselib ${SOURCES}) target_link_libraries (uselib ${PROJECT_LINK_LIBS})
Open up a Terminal. Change directory to the /path/to/workspace/uselib/ folder.
If the build directory does not exist, type in the following to create it.
$ mkdir build
Type in the cmake command with the CMAKE_TOOLCHAIN_FILE variable pointing to the previously created Raspberry Pi toolchain CMake file.
$ cmake -D CMAKE_TOOLCHAIN_FILE=/path/to/workspace/toolchain-rpi.cmake ..
![]() |
The build files are generated |
$ make
![]() |
Compilation messages |
![]() |
Running the Raspberry Pi executable on the Ubuntu PC with QEMU |